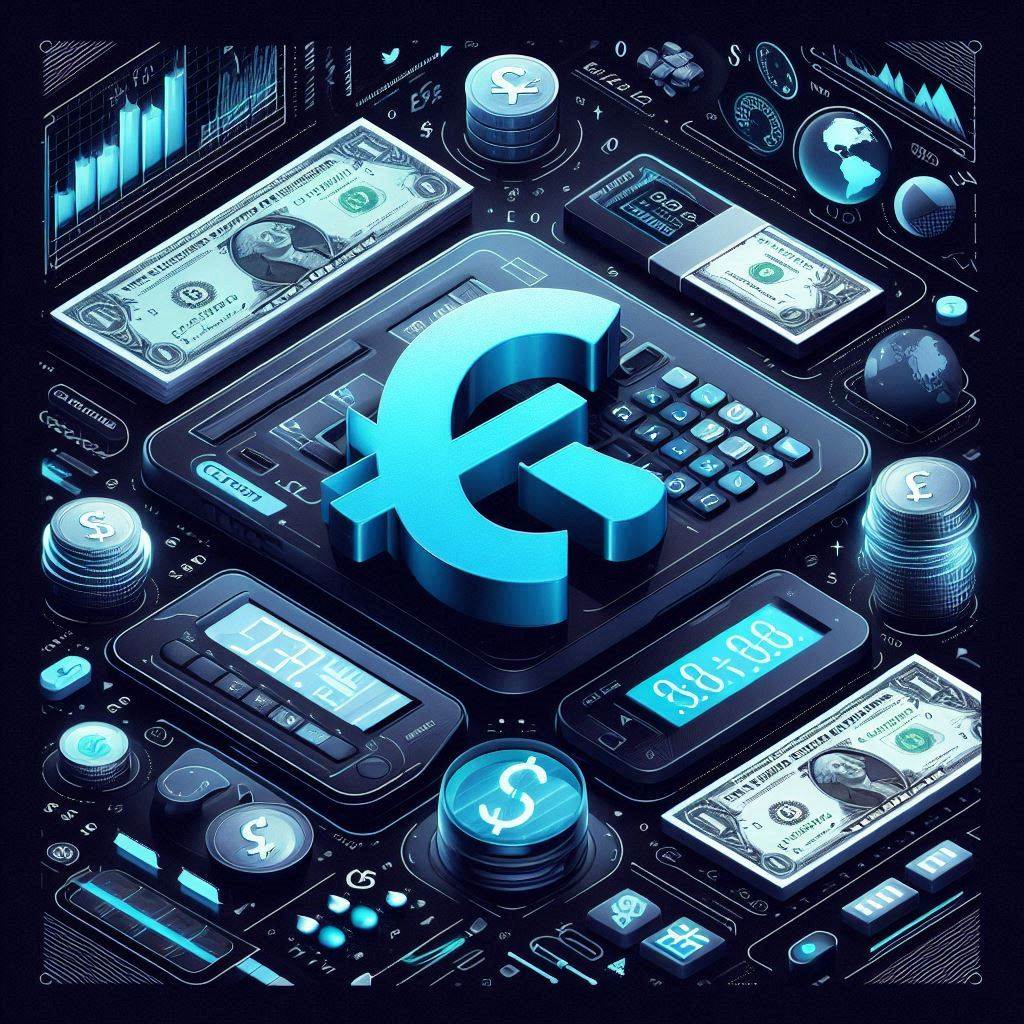
Creating a currency converter app using HTML, CSS, and JavaScript involves building a simple web page that interacts with a currency conversion API to fetch exchange rates. Here’s a step-by-step guide to help you create a basic currency converter.
Step 1: Set Up the HTML
Create an HTML file (index.html
) to define the structure of the app.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Currency Converter</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1>Currency Converter</h1>
<div class="converter">
<div class="input-group">
<input type="number" id="amount" placeholder="Enter amount" />
<select id="from-currency">
<!-- Options will be populated by JavaScript -->
</select>
</div>
<div class="input-group">
<select id="to-currency">
<!-- Options will be populated by JavaScript -->
</select>
<input type="text" id="result" placeholder="Converted amount" readonly />
</div>
<button id="convert">Convert</button>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Step 2: Style the App with CSS
Create a CSS file (styles.css
) to style the app.
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.container {
background-color: white;
padding: 20px;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
text-align: center;
}
h1 {
margin-bottom: 20px;
}
.converter {
display: flex;
flex-direction: column;
gap: 15px;
}
.input-group {
display: flex;
gap: 10px;
justify-content: center;
}
input, select {
padding: 10px;
border-radius: 5px;
border: 1px solid #ddd;
font-size: 16px;
}
button {
padding: 10px 20px;
border: none;
border-radius: 5px;
background-color: #007BFF;
color: white;
font-size: 16px;
cursor: pointer;
}
button:hover {
background-color: #0056b3;
}
Step 3: Add the JavaScript Logic
Create a JavaScript file (script.js
) to handle the logic for fetching exchange rates and performing the conversion.
document.addEventListener('DOMContentLoaded', () => {
const amountInput = document.getElementById('amount');
const fromCurrency = document.getElementById('from-currency');
const toCurrency = document.getElementById('to-currency');
const resultInput = document.getElementById('result');
const convertButton = document.getElementById('convert');
// Replace with your own API key from exchangerate-api.com
const API_KEY = 'Your_api_key';
const API_URL = `https://v6.exchangerate-api.com/v6/${API_KEY}/latest/USD`;
fetch(API_URL)
.then(response => response.json())
.then(data => {
const currencies = Object.keys(data.conversion_rates);
currencies.forEach(currency => {
const option1 = document.createElement('option');
const option2 = document.createElement('option');
option1.value = currency;
option2.value = currency;
option1.textContent = currency;
option2.textContent = currency;
fromCurrency.appendChild(option1);
toCurrency.appendChild(option2);
});
})
.catch(error => console.error('Error fetching exchange rates:', error));
convertButton.addEventListener('click', () => {
const amount = parseFloat(amountInput.value);
const from = fromCurrency.value;
const to = toCurrency.value;
if (isNaN(amount)) {
alert('Please enter a valid amount');
return;
}
fetch(`https://v6.exchangerate-api.com/v6/${API_KEY}/latest/${from}`)
.then(response => response.json())
.then(data => {
const rate = data.conversion_rates[to];
const convertedAmount = (amount * rate).toFixed(2);
resultInput.value = `${convertedAmount} ${to}`;
})
.catch(error => console.error('Error fetching conversion rate:', error));
});
});
Step 4: Obtain an API Key
Sign up for an API key from a currency conversion API provider, such as ExchangeRate-API or Open Exchange Rates.
Replace YOUR_API_KEY_HERE
in the JavaScript file with your actual API key.
Step 5: Run Your App
Open the index.html
file in your web browser to see the currency converter in action.
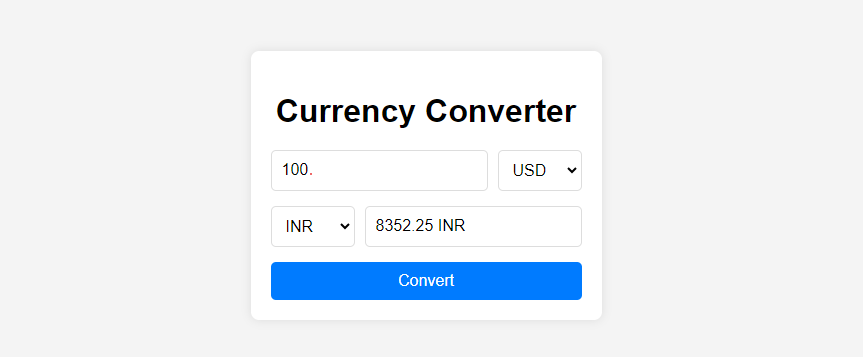
Conclusion
This simple currency converter app built with HTML, CSS, and JavaScript allows users to input an amount and select currencies to convert between. You can enhance this app by adding more features such as historical data, a more sophisticated user interface, or additional currency information.