In PHP, static
and self
are both keywords used to refer to classes and class members, but they have different meanings and behaviors.
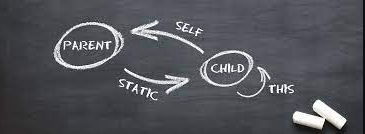
static keyword:
- When used in the context of a class member (property or method),
static
refers to the class itself rather than an instance of the class. - A static property or method belongs to the class itself, and it can be accessed without creating an instance of the class.
- Static properties are shared among all instances of the class, and their values are maintained across different instances.
- Static methods can be called directly on the class without instantiating it.
- Example:
class MyClass {
public static $myStaticProperty = 'Hello';
public static function myStaticMethod() {
echo self::$myStaticProperty;
}
}
echo MyClass::$myStaticProperty; // Output: Hello
MyClass::myStaticMethod(); // Output: Hello
self keyword:
- When used in the context of a class,
self
refers to the class itself and is used to access its own static members (properties and methods). self
is typically used within the class to refer to its own static members.- It does not allow for late static binding, meaning it always refers to the class in which it is used, even in the case of inheritance or overridden methods.
- Example:
class MyClass {
public static $myStaticProperty = 'Hello';
public static function myStaticMethod() {
echo self::$myStaticProperty;
}
}
class AnotherClass extends MyClass {
public static $myStaticProperty = 'World';
}
MyClass::myStaticMethod(); // Output: Hello
AnotherClass::myStaticMethod(); // Output: Hello (not World)
In summary, static
is used to define and access static members of a class, while self
is used to refer to the current class and access its own static members.
[…] self vs static […]