What Are Hooks?
Hooks are a super helpful feature in programming that allow you to extend or change how things work without having to mess with the main code. They’re like little extensions that let you add or tweak functionality, whether you’re building a website, an app, or customizing software. You can think of hooks as ways to “hook into” different parts of a program and change or enhance them.
Why Are Hooks Used?
Hooks are used because they make life easier for developers. Instead of rewriting large portions of code or diving deep into the core system, you can use hooks to:
- Customize functionality to suit your needs.
- Simplify complex tasks like managing state or responding to events.
- Keep your code organized and easier to maintain by separating different pieces of logic.
How Do Hooks Work?
At their core, hooks are like a bridge between the existing code and your custom features. For example, in React, hooks allow you to manage state, handle side effects (like fetching data), and more, all without needing to rely on class-based components. In WordPress, hooks allow you to modify how things work—like adding your custom actions or modifying content—without touching the core files of WordPress itself.
Key Features of Hooks
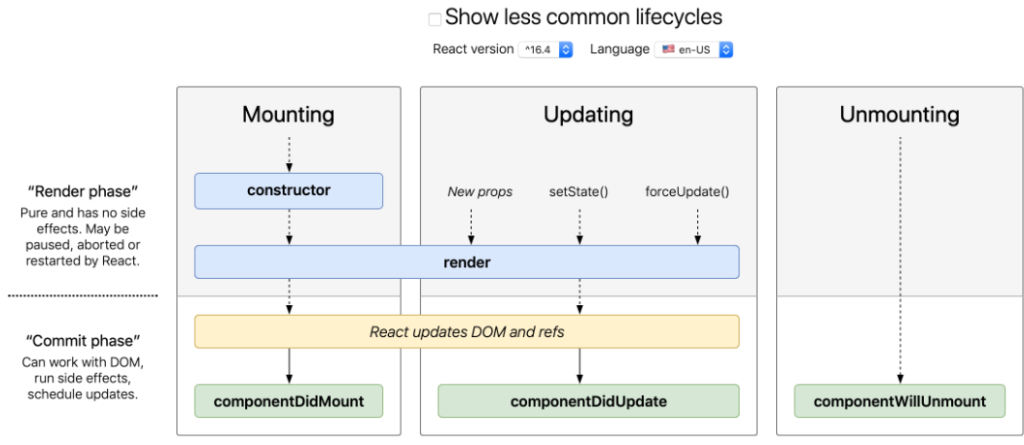
- State Management: In React, the
useState
hook lets you manage and track changes in your app’s state. - Lifecycle Management: The
useEffect
hook helps you run tasks like fetching data when your component loads or updating content when something changes. - Reusability: With custom hooks, you can package up logic and reuse it across different parts of your application.
- Cleaner Code: By using hooks, you avoid the need for bulky class-based components, which makes your code easier to read and maintain.
- Separation of Concerns: Hooks let you keep each piece of functionality focused and modular, which leads to cleaner, more maintainable code.
Main Components of Hooks
- Action Hooks (WordPress): These let you run custom code at specific points in WordPress—such as when a post is published or when a comment is added.
- Filter Hooks (WordPress): These allow you to tweak data before it’s displayed on the site, like changing the title of a post before it’s shown to the user.
- useState (React): Manages state in functional components—think of it like a way to track things like form input or UI status.
- useEffect (React): Lets you handle side effects, such as running a function when the component mounts or when specific data changes.
- useContext (React): Helps you share state or data between components without passing props down manually.
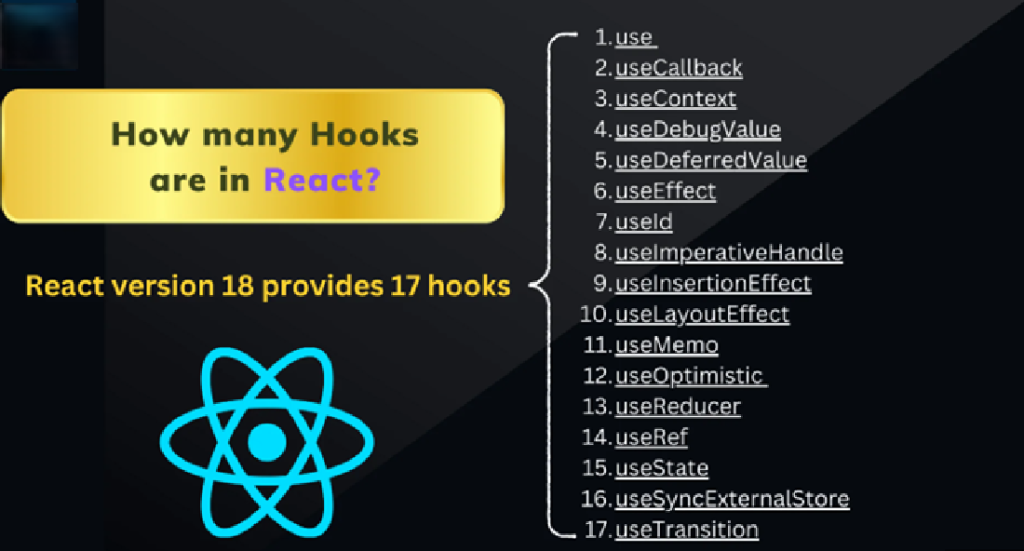
When Should You Use Hooks?
You should reach for hooks:
- When you’re building a React app and need to manage state, handle lifecycle methods, or run side effects.
- When you’re working with WordPress and want to change or add functionality without altering core files.
- Whenever you want a modular, clean way to organize your code into manageable pieces that can be reused across your application.
Benefits of Using Hooks
- Cleaner and Simpler Code: With hooks, you don’t have to write long class-based components. This makes your code easier to understand and maintain.
- Reusability: You can write custom hooks that encapsulate logic you need to reuse across different parts of your app.
- Better Organization: Since hooks allow you to break code into smaller, focused functions, you end up with a better-organized codebase.
- Improved Readability: Smaller, modular code that doesn’t require tons of classes or complicated structures makes your code much more readable.
Challenges or Limitations of Hooks
- Learning Curve: For newcomers or developers used to older paradigms, hooks can be a bit tricky to get the hang of.
- Overusing Hooks: If you’re not careful, using too many hooks or overly complex custom hooks can make your code hard to follow.
- State Management: As your app grows, managing state with hooks alone can get tricky, especially if you don’t use tools like Redux or the Context API.
How to Get Started with Hooks?
- Start Simple: Begin by learning the basics—start with the
useState
anduseEffect
hooks in React. For WordPress, get familiar with how action and filter hooks work. - Practice in Small Projects: Apply hooks in small, simple projects to get the hang of them.
- Use Documentation: Official docs are always a great place to learn more—whether it’s React’s or WordPress’s documentation, they have plenty of examples and explanations.
- Build Custom Hooks: Once you feel comfortable, try creating your own custom hooks for reusable logic.
Alternatives to Hooks
Before hooks were popular, developers used other techniques:
- Class-based Components in React: Before hooks, React used class components for state and lifecycle management.
- Callbacks and Event Listeners in JavaScript: These were used to handle asynchronous actions and event-based programming.
- WordPress Shortcodes: In some cases, shortcodes in WordPress can provide a simpler way to add dynamic content without using hooks.
Real-World Use Cases of Hooks
- React: Companies like Facebook, Instagram, and Airbnb use hooks in their React applications to manage state, effects, and improve performance.
- WordPress: WordPress developers use hooks to build powerful plugins and themes without editing core files. For instance, WooCommerce uses hooks to allow customizations for product displays and checkout processes.
- JavaScript: Many modern web apps use hooks or event listeners for handling interactions like button clicks, form submissions, or server responses in real-time.
By understanding and using hooks, developers can write clean, maintainable, and reusable code for both web and mobile applications. Whether you’re working with React, WordPress, or JavaScript, hooks make your development process more flexible and efficient.
Let me know if you’d like further elaboration or examples!