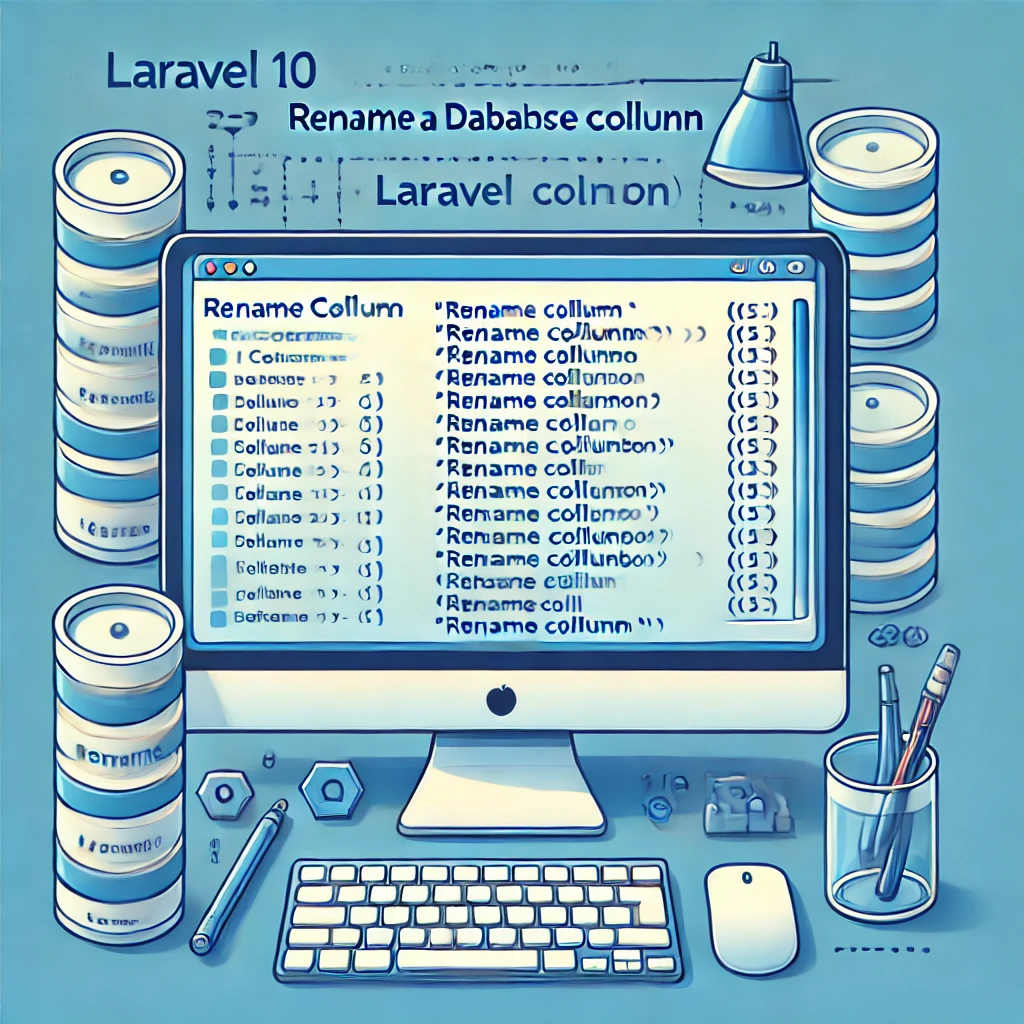
Rename Column in Laravel 10
Introduction
In this tutorial, we’ll learn how to rename a column in a Laravel 10 table without losing any data. Laravel 10 has built-in support for renaming columns, making it a simple and efficient process. Follow the step-by-step guide to seamlessly rename your columns.
Step 1: Create a New Migration
To rename a column, we need to create a new migration file. Run the following Artisan command in your terminal:
php artisan make:migration rename_qrcode_column_in_users_table --table=users
- This command will create a migration file to modify the
users
table where we can specify the column rename operation.
Tip: Replace users
with your table name if you want to rename a column in another table.
Step 2: Modify the Migration File
Next, open the newly created migration file located in the database/migrations
directory. You will find a class named RenameQrcodeColumnInUsersTable
or something similar.
Update the migration file to look like this:
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class RenameQrcodeColumnInUsersTable extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::table('users', function (Blueprint $table) {
$table->renameColumn('Qrcode', 'new_column_name');
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::table('users', function (Blueprint $table) {
$table->renameColumn('new_column_name', 'Qrcode');
});
}
}
Explanation:
- In the
up
method, we’re renaming theQrcode
column tonew_column_name
. - In the
down
method, we’re reversing the change, renamingnew_column_name
back toQrcode
.
Important: Replace new_column_name
with the actual name you want for the column.
Step 3: Run the Migration
Now that the migration is set up, it’s time to execute it. Run the following command:
php artisan migrate
- This will rename the
Qrcode
column tonew_column_name
in yourusers
table without losing any data.
Step 4: Verify the Change
You can verify that the column name has changed successfully by checking the structure of your users
table in your database management tool (e.g., phpMyAdmin, DBeaver, or any other database client).
Troubleshooting Tips
- Ensure that your database version is compatible with the
RENAME COLUMN
operation. MySQL 8.0+ and MariaDB 10.5.2+ support this command. - If you face compatibility issues, consider upgrading your database version.
Why This Method Works
In Laravel 10, the renameColumn
method is supported out of the box, meaning you don’t need additional packages like doctrine/dbal
. This feature ensures a smooth column rename process, retaining all existing data.
Conclusion
Renaming columns in Laravel 10 is a straightforward process, thanks to its built-in support. By following this guide, you can efficiently rename any column in your database without losing data.