This guide combines everything from my two previous responses into a clear and structured tutorial you can follow or share with your team
๐ The Problem
When running:
npm install @vitejs/plugin-react
You saw:
npm ERR! code EACCES
npm ERR! syscall mkdir
npm ERR! path /usr/sbin/.npm
npm ERR! errno -13
npm ERR! Your cache folder contains root-owned files...
This happens because:
- npm is using a global cache directory belonging to root.
- Files in
/root/.npm
or/usr/sbin/.npm
are not writable by your current user. - This happens if you ever ran
sudo npm install
directly.
๐ Goal
We will:
- Set npm cache and global directory to your userโs home folder (recommended).
- Fix permissions for
.npm
folders. - Update Laravel’s
Process
command to avoid this issue in your Laravel installer.
๐ ๏ธ Step 1 – Check Current npm Cache Path
Run:
npm config get cache
If you see:
/root/.npm
This is the root cause of the error โ npm is using a root-owned directory, even when running as a normal user.
๐ ๏ธ Step 2 – Set npm Global Directory to Your User Folder
Run:
mkdir -p ~/.npm-global
npm config set prefix '~/.npm-global'
npm config set cache '~/.npm-global/_cache'
This tells npm to:
- Install global packages to
~/.npm-global
- Use
~/.npm-global/_cache
for cache files (under your home directory)
๐ ๏ธ Step 3 – Add npm Global Directory to PATH
You need to add the new npm global bin
directory to your PATH so globally installed packages can be run.
Run this command to add it to ~/.bashrc
(for Ubuntu/Debian):
echo 'export PATH=~/.npm-global/bin:$PATH' >> ~/.bashrc
source ~/.bashrc
๐ ๏ธ Step 4 – Fix Ownership of Old npm Folders
If you previously ran npm as sudo
, you need to fix permissions for existing .npm
folders:
sudo chown -R $USER:$USER ~/.npm ~/.npm-global
This ensures all files in these folders belong to your normal user.
๐ ๏ธ Step 5 – Verify It Worked
Run:
npm config get cache
Expected output:
/home/your-username/.npm-global/_cache
This confirms npm is now using a user-owned directory.
๐ ๏ธ Step 6 – Test npm Manually
Test:
npm install @vitejs/plugin-react
npm install react-places-autocomplete
npm run build
If no errors, you are all set!
๐ ๏ธ Step 7 – Fix Laravelโs InstallController (Optional)
If you have a Laravel installer that runs npm commands using:
$process = new Process(['npm', 'install', '@vitejs/plugin-react']);
You can make sure Laravel uses the correct PATH:
$env = array_merge($_ENV, [
'PATH' => getenv('PATH') . ':' . getenv('HOME') . '/.npm-global/bin',
]);
$process = new Process(['npm', 'install', '@vitejs/plugin-react'], base_path(), $env);
This ensures the npm
command will use your user-specific npm global folder, avoiding future permission errors.
All process in image
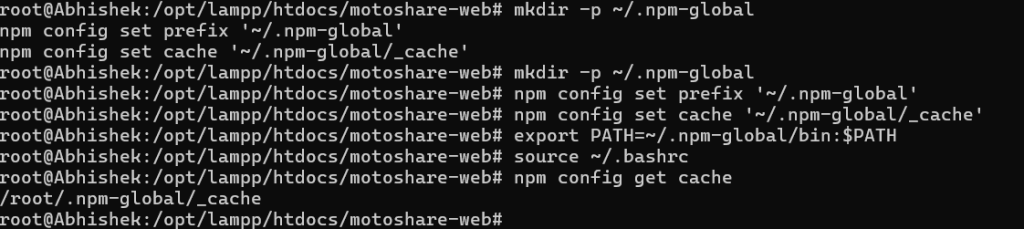
โ
Final Summary – All Commands Together
Hereโs the full command list you can copy-paste to fix this issue:
# Check current npm cache location
npm config get cache
# Create and configure user-owned npm global folder
mkdir -p ~/.npm-global
npm config set prefix '~/.npm-global'
npm config set cache '~/.npm-global/_cache'
# Add npm global bin to PATH
echo 'export PATH=~/.npm-global/bin:$PATH' >> ~/.bashrc
source ~/.bashrc
# Fix ownership if needed
sudo chown -R $USER:$USER ~/.npm ~/.npm-global
# Verify cache location
npm config get cache
# Test npm commands
npm install @vitejs/plugin-react
npm install react-places-autocomplete
npm run build
๐ฅ Optional – Full Bash Script (install-npm-fix.sh)
If you want, you can create a bash script so you can reuse it anytime.
Create install-npm-fix.sh
:
nano install-npm-fix.sh
Paste this inside:
#!/bin/bash
echo "Fixing npm EACCES and root cache issues..."
mkdir -p ~/.npm-global
npm config set prefix '~/.npm-global'
npm config set cache '~/.npm-global/_cache'
echo 'export PATH=~/.npm-global/bin:$PATH' >> ~/.bashrc
source ~/.bashrc
sudo chown -R $USER:$USER ~/.npm ~/.npm-global
echo "npm cache location:"
npm config get cache
echo "npm setup complete!"
Make it executable:
chmod +x install-npm-fix.sh
Run it anytime with:
./install-npm-fix.sh
โ Conclusion
This solves:
npm ERR! code EACCES
npm ERR! path /root/.npm
- Ensures npm is 100% safe to use without sudo.
- Works for LAMPP, Ubuntu, Debian, or any Linux server.