Arrow function
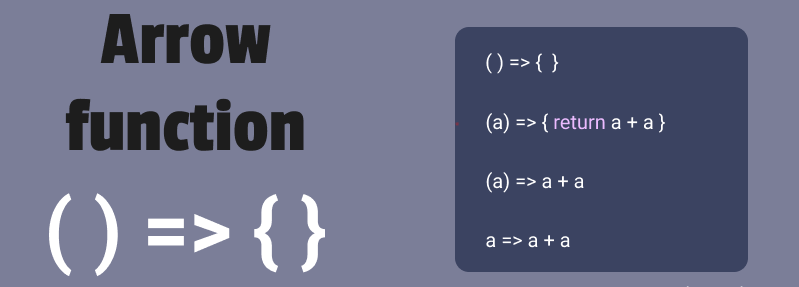
Arrow function is one of the features introduced in the ES6 version of JavaScript. It allows you to create functions in a cleaner way compared to regular functions. For example,
you can define a simple arrow function as follows:
const greet = () => {
console.log("Hello!");
};
greet(); // Output: Hello!
In this example, the arrow function greet
does not take any parameters and simply logs “Hello!” to the console.
Here’s an example of an arrow function with a parameter:
const square = (num) => {
return num * num;
};
console.log(square(5)); // Output: 25
The arrow function square takes a num
parameter, multiplies it by itself, and returns the result.